Create Hierarchical Queries in Oracle SQL with CONNECT BY PRIOR
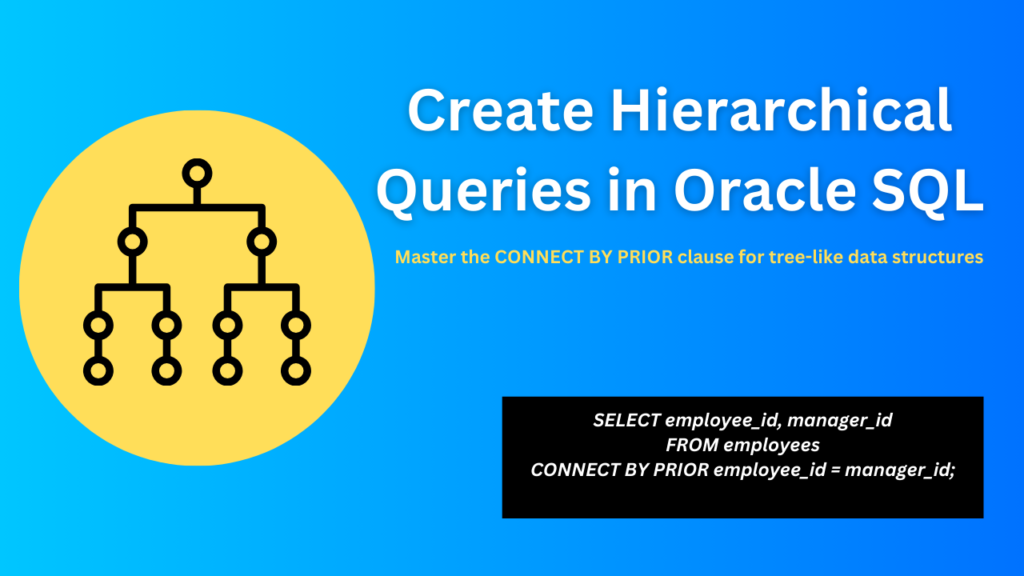
The CONNECT BY PRIOR clause is a powerful feature in Oracle SQL for hierarchical queries, allowing users to navigate and retrieve data with a parent-child relationship within the same table. Here’s an in-depth look at this feature, its syntax, usage, and examples.
Hierarchical queries are used to retrieve data based on hierarchical relationships, such as organizational structures, bill of materials, family trees, etc. The CONNECT BY PRIOR clause enables these queries by specifying how rows are related in a parent-child hierarchy.
Syntax
The basic syntax for a hierarchical query using CONNECT BY PRIOR is:
SELECT column1, column2, ... FROM table START WITH condition1 CONNECT BY PRIOR condition2;
- START WITH: Defines the root(s) of the hierarchy. This condition determines which rows are the starting points.
- CONNECT BY PRIOR: Defines the hierarchical relationship between parent and child rows. The PRIOR keyword is used to indicate the parent row in the relationship.
Example
Consider an Employee table with the following data structure:
employee_id | employee_name | manager_id |
---|---|---|
1 | John Doe | NULL |
2 | Jane Smith | 1 |
3 | Michael Lee | 1 |
4 | Alice Wong | 2 |
5 | Bob Brown | 6 |
6 | David Lee | NULL |
To retrieve the hierarchical structure of this table, you can use below query.
SELECT employee_id, employee_name, manager_id FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id;
In this context, START WITH manager_id IS NULL indicates that the hierarchy’s roots are employees who do not have a manager.
CONNECT BY PRIOR employee_id = manager_id establishes the parent-child relationship by matching the employee_id of a parent to the manager_id of a child.
Result:
employee_id | employee_name | manager_name |
---|---|---|
1 | John Doe | NULL |
2 | Jane Smith | 1 |
4 | Alice Wong | 2 |
3 | Michael Lee | 1 |
6 | David Lee | NULL |
5 | Bob Brown | 6 |
Key Pseudo-columns
Pseudo-columns are special columns provided by the Oracle database that are not stored in the table but can be used in SQL queries to return specific values. Pseudo-columns enhance the functionality of hierarchical queries in Oracle SQL. They provide valuable insights into the structure and traversal of hierarchical data, making it easier to understand and manipulate complex relationships within a table. The following key pseudo-columns are commonly used with CONNECT BY PRIOR for hierarchical queries.
LEVEL:
It indicates the level of the node in the hierarchy. It is useful for understanding the depth of each row in the hierarchical structure. The root level starts at 1 and each subsequent level increments by 1 for each child node.
Oracle SQL automatically calculates the LEVEL for each row when you perform a hierarchical query using the CONNECT BY PRIOR clause. The calculation is based on the hierarchical relationship defined by the CONNECT BY PRIOR condition.
Example:
SELECT employee_id, employee_name, manager_id, LEVEL FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id;
Result:
employee_id | employee_name | manager_id | LEVEL |
---|---|---|---|
1 | John Doe | NULL | 1 |
2 | Jane Smith | 1 | 2 |
4 | Alice Wong | 2 | 3 |
3 | Michael Lee | 1 | 2 |
6 | David Lee | NULL | 1 |
5 | Bob Brown | 6 | 2 |
Uses:
- Indentation in Reports: Use the LEVEL to indent hierarchical data for better readability.
SELECT LPAD(' ', LEVEL * 2) || employee_name AS indented_name FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id;
This query will produce an indented list of employee names according to their level in the hierarchy.
- Filtering by Depth: Retrieve rows up to a certain depth in the hierarchy.
SELECT employee_id, employee_name, manager_id, LEVEL FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id WHERE LEVEL <= 2;
This query fetches only those employees who are within two levels from the root.
- Aggregations by Level: Perform aggregations based on the level.
SELECT LEVEL, COUNT(*) AS num_employees FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id GROUP BY LEVEL;
This query counts the number of employees at each level in the hierarchy.
CONNECT_BY_ISLEAF
It is used to identify whether a particular row is a leaf node in hierarchical queries. A leaf node is a node that does not have any children, meaning it is at the end of a branch in the hierarchy. Returns 1 if the node is a leaf node and 0 if not.
It is particularly useful when you need to distinguish between leaf nodes and non-leaf nodes in the result set of a hierarchical query. This can be helpful for reporting, analysis, or any scenario where the differentiation between terminal nodes and intermediate nodes is necessary.
SELECT employee_id, employee_name, manager_id, CONNECT_BY_ISLEAF AS is_leaf FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id;
Result:
employee_id | employee_name | manager_id | is_leaf |
1 | John Doe | 0 | |
2 | Jane Smith | 1 | 0 |
4 | Alice Wong | 2 | 1 |
3 | Michael Lee | 1 | 1 |
6 | David Lee | 0 | |
5 | Bob Brown | 6 | 1 |
Uses:
- Filtering Leaf Nodes: To retrieve only the leaf nodes from the hierarchy.
SELECT employee_id, employee_name, manager_id, LEVEL FROM employees START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id HAVING CONNECT_BY_ISLEAF = 1;
- Aggregating Data: Summarize data specifically for leaf nodes.
SELECT manager_id, COUNT(*) AS num_leaf_employees FROM employees START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id HAVING CONNECT_BY_ISLEAF = 1 GROUP BY manager_id;
CONNECT_BY_ROOT
It returns the root node of each row in the hierarchy. It is useful for showing the top-most parent of each row in the hierarchy.
SELECT employee_id, employee_name, manager_id, CONNECT_BY_ROOT employee_name AS root_name FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id;
Result:
employee_id | employee_name | manager_id | root_name |
1 | John Doe | John Doe | |
2 | Jane Smith | 1 | John Doe |
4 | Alice Wong | 2 | John Doe |
3 | Michael Lee | 1 | John Doe |
6 | David Lee | David Lee | |
5 | Bob Brown | 6 | David Lee |
Uses:
- Multiple Root Columns: You can use CONNECT_BY_ROOT with multiple columns to retrieve various root-level details.
- Complex Hierarchies: In more complex hierarchical structures, CONNECT_BY_ROOT helps in tracing the origin of each node, providing a clearer understanding of the hierarchy.
SYS_CONNECT_BY_PATH
Returns the path from the root to the current row as a string. It is useful in visualizing the entire hierarchy and understanding the sequence of nodes from the top to any given node in the tree.
SELECT employee_id, employee_name, SYS_CONNECT_BY_PATH(employee_name, ' -> ') AS path FROM employee START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id;
Result:
employee_id | employee_name | path |
1 | John Doe | -> John Doe |
2 | Jane Smith | -> John Doe -> Jane Smith |
4 | Alice Wong | -> John Doe -> Jane Smith -> Alice Wong |
3 | Michael Lee | -> John Doe -> Michael Lee |
6 | David Lee | -> David Lee |
5 | Bob Brown | -> David Lee -> Bob Brown |
Uses:
- Display the full hierarchy path of a node.
- Understand the ancestry of a node within the hierarchical structure.
Advanced Examples
Sibling Order
Sibling order refers to the ordering of nodes that share the same parent in a hierarchical query. The ORDER SIBLINGS BY clause is used to sort siblings based on specified columns. This feature ensures that the hierarchical structure is maintained while sorting siblings at each level according to the given criteria.
Syntax:
SELECT column1, column2, ... FROM table START WITH condition1 CONNECT BY PRIOR condition2 ORDER SIBLINGS BY column3, column4, ...;
ORDER SIBLINGS BY: Specifies the columns by which siblings should be ordered. Multiple columns can be listed, separated by commas. In this case, column3, column4, …
Example:
SELECT employee_id, employee_name, manager_id, LEVEL FROM employees START WITH manager_id IS NULL CONNECT BY PRIOR employee_id = manager_id ORDER SIBLINGS BY employee_name;
ORDER SIBLINGS BY: Specifies the columns used to order siblings. In this case, using employee_name sorts employees under the same manager by their names.
Avoiding Cycles
A cycle occurs in a hierarchical query when there is a circular relationship in the data structure. For example, an employee directly or indirectly reporting to themselves, or a parent node having a descendant that eventually points back to it through other nodes.
To prevent cycles in Oracle SQL hierarchical queries, you can use the NOCYCLE keyword with CONNECT BY PRIOR. This keyword instructs Oracle to stop traversing a path if a cycle is detected, ensuring that the query does not enter an infinite loop.
Syntax:
SELECT ... FROM ... START WITH ... CONNECT BY NOCYCLE PRIOR ...
Example:
SELECT employee_id, employee_name, manager_id, LEVEL FROM employees START WITH manager_id IS NULL CONNECT BY NOCYCLE PRIOR employee_id = manager_id;
Benefits:
- Prevents Infinite Loops: Stops the query from endlessly traversing cycles in the data structure.
- Ensures Query Integrity: Provides correct and reliable hierarchical data retrieval by avoiding erroneous results caused by cycles.
Performance
When using CONNECT BY PRIOR, it is essential to consider performance implications, particularly with large datasets or complex hierarchies. Here is a detailed look at potential performance issues and tips to address them.
Performance Implications
- Resource Intensive: Hierarchical queries can consume significant CPU and memory resources, particularly with deep or complex hierarchies.
- Execution Time: The query execution time increases with the depth and breadth of the hierarchy, as each level adds more joins and computations.
- Data Volume: Large datasets can further impact performance, as more data needs to be processed and joined.
- Recursive Nature: The recursive processing required for hierarchical queries can lead to longer execution times compared to flat queries.
Optimization Tips
- Indexing: Ensure appropriate indexing on columns used in the CONNECT BY PRIOR clause (e.g., employee_id and manager_id in the example above). This can significantly improve query performance by reducing the search space.
- Filter Early: Use the START WITH clause to limit the initial set of rows processed. Filtering early reduces the number of rows that need to be recursively processed.
- Avoiding Cycles: Use the NOCYCLE keyword to prevent infinite loops if the data contains circular references. This avoids excessive CPU usage and potential query failures.
- Pseudo-Columns: Use pseudo-columns like LEVEL, CONNECT_BY_ISLEAF, and SYS_CONNECT_BY_PATH wisely to understand your data better.
- Materialized Views: For frequently accessed hierarchical data, consider using materialized views to store the results of hierarchical queries. This can drastically reduce query execution time.
Difference between Self-Joins and CONNECT BY PRIOR
- Self-Joins are for relating rows within the same table, while CONNECT BY PRIOR is specifically for hierarchical queries.
- Self-joins use standard join syntax, whereas CONNECT BY PRIOR requires specific hierarchical query syntax.
- Self-joins produce flat result sets, whereas CONNECT BY PRIOR generates hierarchical structures with levels and paths.
- Self-joins is a general SQL feature, while CONNECT BY PRIOR is specific to Oracle SQL.
Summary
In this post, we explored the powerful CONNECT BY PRIOR clause in Oracle SQL for querying hierarchical data. We covered its syntax and provided examples. We also discussed useful pseudo-columns like LEVEL, CONNECT_BY_ISLEAF, CONNECT_BY_ROOT, and SYS_CONNECT_BY_PATH, which add flexibility and detail to hierarchical queries. Additionally, we examined the performance issues associated with CONNECT BY PRIOR and shared optimization techniques to address these challenges. You can find all the SQL queries we discussed throughout this article at this link.
Leave a Reply